We Make it Really Simple
So you can focus on UX - not the back end.
Get Collection Method
// Install with: npm install @tatumio/tatum
const { TatumSDK, Network } = require("@tatumio/tatum");
(async () => {
try {
const tatum = await TatumSDK.init({ network: Network.ETHEREUM });
const nfts = await tatum.nft.getNftsInCollection({
collectionAddress: '0xBC4CA0EdA7647A8aB7C2061c2E118A18a936f13D', // replace with your collection
});
console.log(nfts.data);
} catch (error) {
console.error("Error fetching NFT collection:", error);
}
})();
tatum.destroy()
See All NFTs in a Collection
Effortlessly manage NFT collections with Tatum. Quickly and reliably retrieve data for every unique digital asset to build a smooth app experience.
Instantly Trace NFT History
From the moment it minted to the moment it sold - trace the history for each NFT instantly and reliably with simple to use lean code.
Get All NFT Transactions Method
// Install with: npm install @tatumio/tatum
const { TatumSDK, Network } = require("@tatumio/tatum");
(async () => {
try {
const tatum = await TatumSDK.init({ network: Network.ETHEREUM });
const txs = await tatum.nft.getAllNftTransactions({
tokenId: '14721',
tokenAddress: '0xccb9d89e0f77df3618eec9f6bf899be3b5561a89', // replace with your collection
});
console.log(txs.data);
} catch (error) {
console.error("Error fetching wallet balance:", error);
}
})();
tatum.destroy()
Create a Collection Method
// Install with: npm install @tatumio/tatum
const { TatumSDK, Network } = require("@tatumio/tatum");
(async () => {
try {
const tatum = await TatumSDK.init({ network: Network.ETHEREUM });
const txs = await tatum.nft.createNftCollection({
name: 'My NFT Collection,
symbol: 'MyNFT'
owner: '0x727ea45b2eb6abb2badd3dc7106d146e0dc0450d', // replace with your address
});
console.log(txs.data.txId);
// 0x8e564406701caab6258501c794f5c1eece380f673be99b561d626c3d8d81b202
const collectionAddress = await tatum.rpc.getContractAddress(tx.data.txId);
console.log(collectionAddress);
// 0x876977006988ce590e219f576077459a49c7318a
} catch (error) {
console.error("Error creating NFT collection:", error);
}
})();
tatum.destroy()
Build an NFT
Collection
Tatum provides the method for the most streamlined and widely used way of minting entire NFT collections.
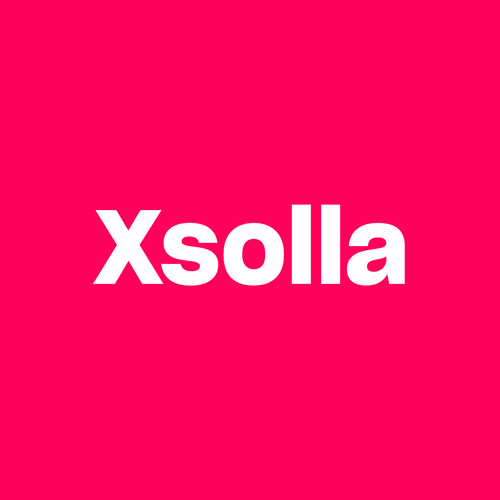
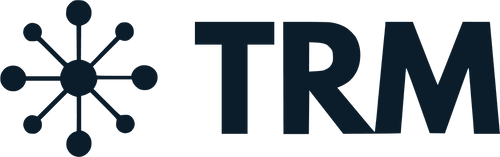
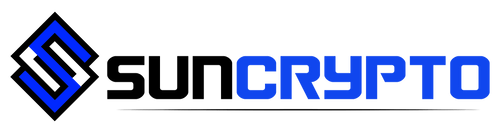


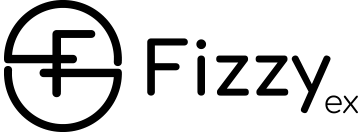
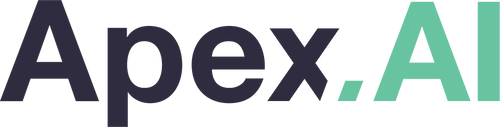
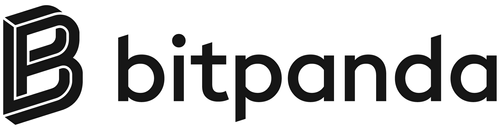

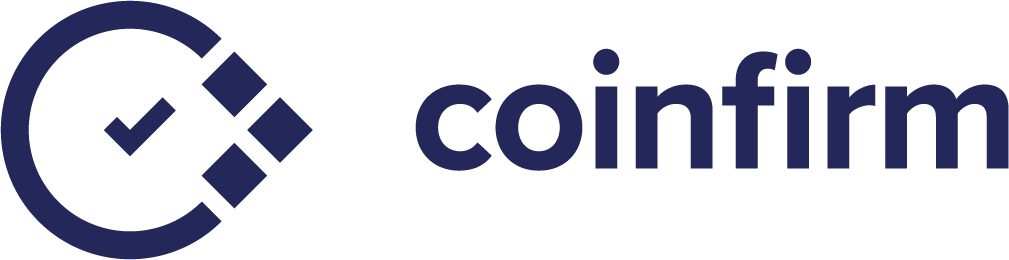


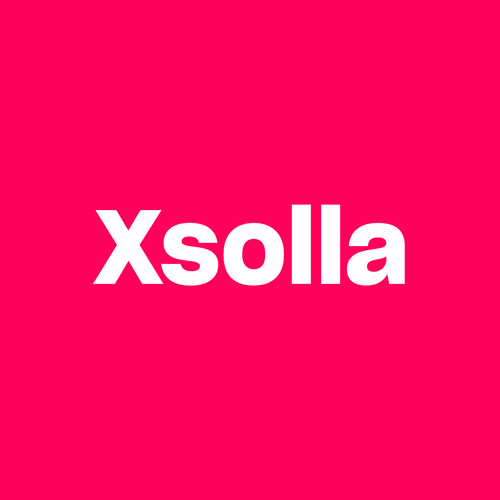
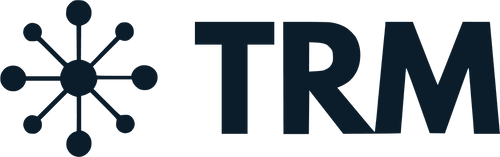
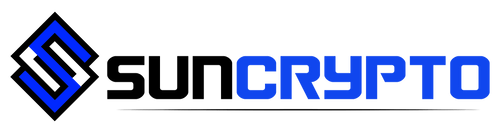


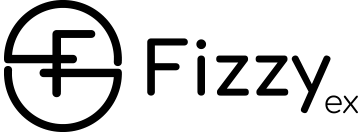
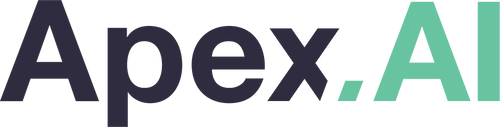
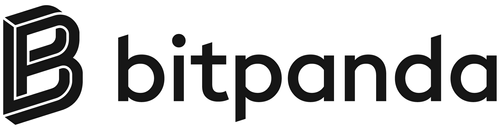

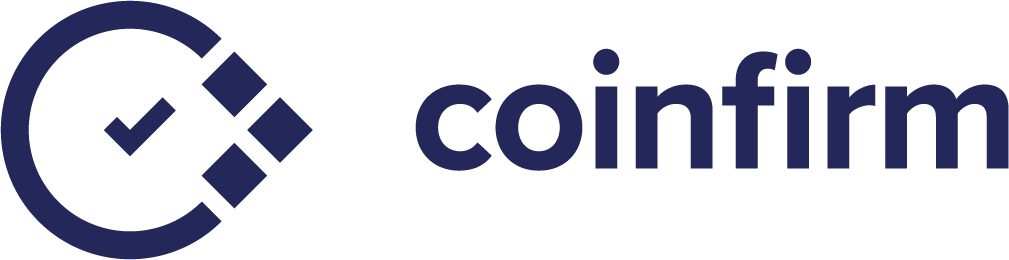


What Devs Say About Us

"Tatum helps us build robust product solutions in a fraction of the time."
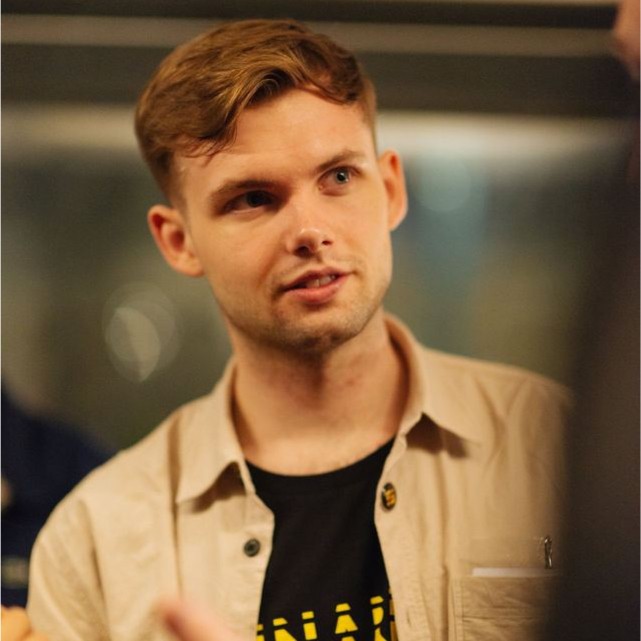
Zac Barron
Product Lead at Binance

"Building a product in the crypto space is hard. Making it work reliably across chains - even harder. Tatum helped us meet our deadline."

David Spitzer-Dulagan
CTO at Limewire
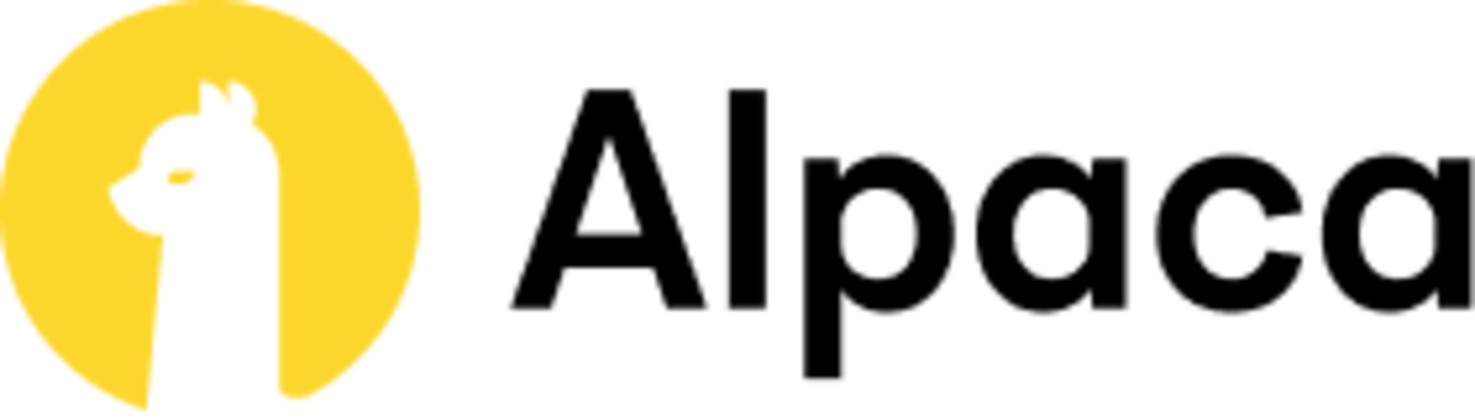
"Tatum accelerates our web3 development."
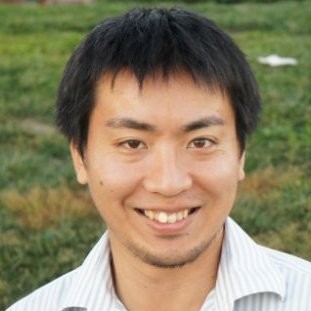
Hitoshi Harada
Co-founder & CPO at Alpaca
Access All Tatum Tools
A powerful SDK, lightning-fast RPC nodes, faucets and a whole lot more - for free.
Sign Up.png)